ArrayList in c#
"ArrayList is a class of Collections^ ,and it's a data structure
that store collection of data just like an array". But it's is little
bit different than ArrayList See the detail difference between Array and
ArrayList at bottom of this page.
EXAMPLE OF ARRAYLIST
1. How to declare ArrayList in C#
To declare an ArrayList first
step to add the directive System. Collection, and what have to do is we have to
the instantiate the instance of ArrayList as we did to the class instance with the help of new keyword see the
example
using System.Collections;
static void Main()
{
ArrayList List =
new ArrayList ();
// the name of ArrayList
will be 'List' or you can chose your own name
}
2. How to add item/values into an Arraylist in C#
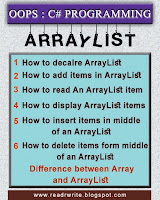 |
arraylist in c# |
With the a
help of
Add
keyword we will add object type data in ArrayList
See it
static void Main()
{
ArrayList List =
new ArrayList ();
List.Add(100);
List.Add("Jhony");
List.Add('a');
}
3. How to read an ArrayList in C#
Like the old process but little
bit change we have to use the Add keyword like this
static void Main()
{
ArrayList List =
new ArrayList();
Console.WriteLine("Enter items into ArrayList");
List.Add(Console.ReadLine());
}
4. How to write and display the output of an ArrayList in C#
Because of
the ArrayList we will use foreach
loop to print the ArrayList
static void Main()
{
ArrayList List =
new ArrayList();
Console.WriteLine("Enter items into ArrayList");
List.Add(Console.ReadLine());
|
array vs arraylist |
List.Add(100);
List.Add("Jhony");
List.Add('a');
Console.Write("Array items are ");
foreach (object obj in List)
Console.Write(" " + obj);
Console.ReadKey();
}
5. How to insert item/values in the middle of ArrayList
For this
you to specify the index number at the place we want to add the item and by
using insert keyword we will insert at any point. Like suppose the above program
I want to add 150 in between the "Jhony" and 'a' thus we have to add
at index number 3 because index start at zero let do it.
static void Main()
{
ArrayList List =
new ArrayList();
Console.WriteLine("Enter items into ArrayList");
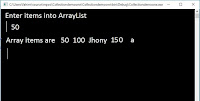 |
array to arraylist |
List.Add(Console.ReadLine());
List.Add(100);
List.Add("Jhony");
List.Add('a');
List.Insert(3,
150);
foreach (object obj in List)
Console.Write(" " + obj);
Console.ReadKey();
}
6.
How to delete item from middle of ArrayList
By
use of either Remove
keyword and specify the 'object obj'. Or RemoveAt keyword by specifying index number. Suppose above
program I want to delete "Jhony" we have two ways
First (Remove keyword and specify
the 'object obj')
static void Main()
{
ArrayList List =
new ArrayList();
Console.WriteLine("Enter items into ArrayList");
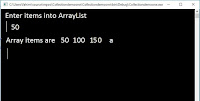 |
array to arraylist |
List.Add(Console.ReadLine());
List.Add(100);
List.Add("Jhony");
List.Add('a');
List.Insert(3,
150);
List.Remove("Jhony");
foreach (object obj in List)
Console.Write(" " + obj);
Console.ReadKey();
}
Second (RemoveAt keyword by specifying
index number)
static void Main()
{
ArrayList List =
new ArrayList();
Console.WriteLine("Enter items into ArrayList");
List.Add(Console.ReadLine());
List.Add(100);
List.Add("Jhony");
List.Add('a');
List.Insert(3,
150);
List.RemoveAt(4);
foreach (object obj in List)
Console.Write(" " + obj);
Console.ReadKey();
}